Cashier Mode
VIP Preferred and VIP Online can also be integrated into the operator's mobile app cashier page. It is invoked the same way as the standard VIP Web Component with the addition of a parameter to specify the smaller Cashier Mode view. See the Invoke Web Component page for more info.
The Cashier Mode is only intended for existing VIP Connect patrons. The operator app can check if a patron has an existing account using the account inquiry API endpoint.
Example UI
The section inside the green box is the VIP Connect Cashier Mode. Outside the green box is an example view of the operator's mobile app cashier page.
Suggested Process Flow
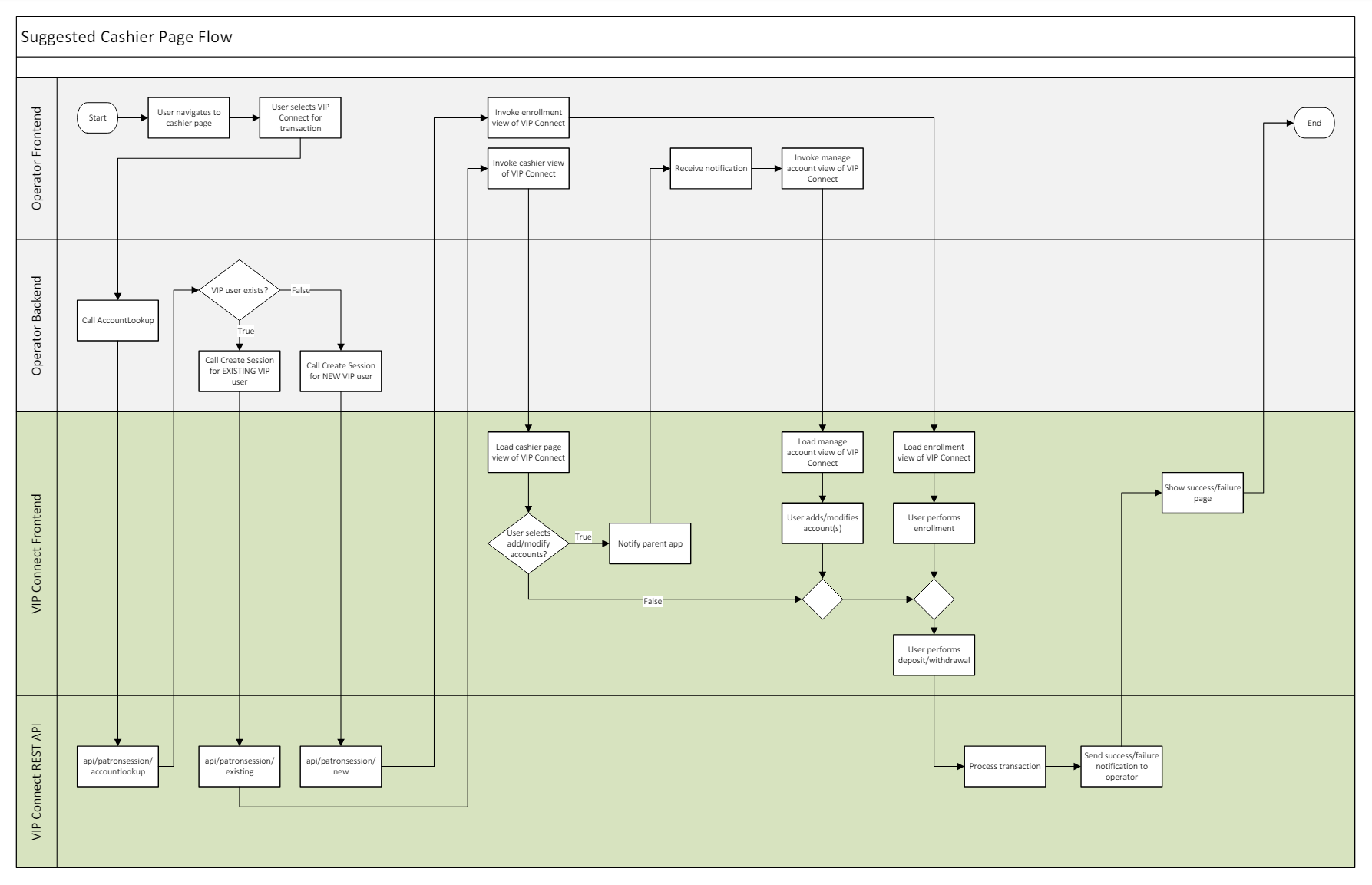
Integrating Cashier Mode in Native apps
When integrating a VIP Connect Cashier Mode in your app, there are additional steps that need to be performed to allow the user to add and manage their bank account list within the view. Your app will need to listen for a JavaScript callback event that may be fired, and reopen the VIP Connect view in a fullscreen mode when the callback is triggered.
iOS Integration
Listening for the request to launch in fullscreen
Configure some object to implement the WKScriptMessageHandlerWithReply protocol, and have that object launch in full screen mode when the "opensdk" message body is found:
class SampleScriptListener: NSObject, WKScriptMessageHandlerWithReply {
public func userContentController(_ userContentController: WKUserContentController, didReceive message: WKScriptMessage, replyHandler: @escaping (Any?, String?) -> Void) {
if (message.body as? String) == "opensdk" {
// Launch full screen view
// for example,
//
// myDelegate.onFullScreenRequested()
return
}
replyHandler(nil, nil)
}
}
Then set that object as the WKWebview's script message handler with the name "NativeBridge":
yourWKWebview.configuration.userContentController.addScriptMessageHandler(SampleScriptListener(), contentWorld: .page, name: "NativeBridge")
The message listener does not need to be a new class, it could also be implemented as an extension on any appropriate object your app is using.
Launching the view in fullscreen
After hearing the request to launch VIP Connect in a full screen view, have your ViewController show a full screen webview, and have that webview relaunch the VIP Connect SDK.
Your app can create and launch the full screen webview however you wish; one simple way to do it would be to present a simple UIViewController that contains only a full screen webview, and have that UIViewController launch the correct url on load:
class ViewController: UIViewController {
// ...
private func onFullScreenRequested() {
let vc = AFullScreenWebViewController()
vc.initialURL = URL(string: "\(Config.VIPConnectHost)?mode=\(transactionType)#\(currentSession)")
self.show(vc, sender: self)
}
// ...
}
When relaunching the VIP Connect session, it is important to use the same session ID as the one that was used to launch the smaller
Cashier Mode view, and not create a new session ID. You should also remove the view=cashier
parameter when opening the session in
full screen mode.
With these steps complete, if the user tries to add or manage an account in Cashier Mode view, the app will reopen VIP Connect in a larger view, and the user will be able to continue their flow.
Android Integration
Listening for the request to launch in fullscreen
Create a class to serve as a JavaScriptInterface (or use an existing class, if you have one) and add the following method to it:
internal class JavaScriptInterface(
private val webView: WebView,
private val fullScreenHandler: () -> Unit,
) {
@JavascriptInterface
fun consumeWindowMessage(name: String?, payload: String?) {
if (payload == "opensdk") {
webView.context.findActivity()?.runOnUiThread {
fullScreenHandler()
}
}
}
}
Then set that object as the WebView's JavaScriptInterface with the name "Android":
@SuppressLint("SetJavaScriptEnabled")
theWebview.settings.javaScriptEnabled = true
theWebview.settings.domStorageEnabled = true
val jsNativeInterface = JavaScriptInterface(
webView = theWebview,
fullScreenHandler = { ... }
)
addJavascriptInterface(jsNativeInterface, "Android")
Additionally, add this code to inject a JavaScript event listener into the VIP Connect pages:
theWebview.webViewClient = object : WebViewClient() {
override fun onPageFinished(view: WebView?, url: String?) {
super.onPageFinished(view, url)
evaluateJavascript("""
window.addEventListener("message", (e) => { window.Android.consumeWindowMessage(e.name, e.data); });
""",
null,
)
onLoadingChange(false)
}
}
Launching the view in fullscreen
After hearing the request to launch VIP Connect in a full screen view, have your Activity show a full screen webview, and have that webview relaunch the VIP Connect SDK.
Your app can create and launch the full screen webview however you wish; one simple way to do it would be to present a simple Fragment that contains only a full screen webview, and have that Fragment launch the correct url on load.
When relaunching the VIP Connect session, it is important to use the same session ID as the one that was used to launch the smaller
Cashier Mode view, and not create a new session ID. You should also remove the view=cashier
parameter when opening the session in
full screen mode.
With these steps complete, if the user tries to add or manage an account in Cashier Mode view, the app will reopen VIP Connect in a larger view, and the user will be able to continue their flow.